프로젝트 환경
- JAVA 11
- IDE : IntelliJ
1. https://start.spring.io/ 에 접속하여 프로젝트 생성
*What is Gradle, Maven?
: 프로젝트에 필요한 플러그인, 라이브러리들을 설치하고 빌드하기까지의 과정을 편리하게 해주는 tool 이라고 보면 된다.
(참고로 요즘 추세는 Gradle 이라고 한다..우리 회사는 아직까지는 Maven을 사용하고 있다...ㅎㅎ)
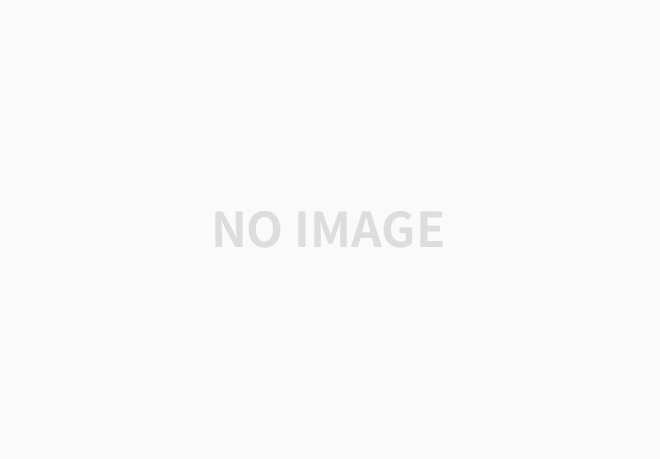
Dependencies는 웹 프로젝트이므로 Spring Web과, HTML을 웹 브라우저에 띄울수 있도록 해주는 템플릿 엔진, Thymeleaf를 설치해주었다.
Generate 버튼을 누르면, 압축파일이 다운로드 받아진다. 압축을 풀고, IDE로 해당 프로젝트를 연다.
2. 프로젝트 구조 보기
ㄴsrc
ㄴmain
ㄴjava //실제 java 소스, 패키징 파일
ㄴresources //java 외 properties 설정파일, xml, html,..
ㄴtest //테스코드
ㄴbuild.gradle //springboot에서 제공하는 설정 파일
build.gradle 파일 살펴보기
plugins {
id 'java'
id 'org.springframework.boot' version '2.7.5'
id 'io.spring.dependency-management' version '1.0.15.RELEASE'
}
group = 'com.example' //프로젝트 생성시 설정한 도메인명
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '11' //java 버전
repositories {
mavenCentral() //dependencies 파일들을 설치하는 곳
}
dependencies { //프로젝트 생성시 설치한 dependencies 명시
implementation 'org.springframework.boot:spring-boot-starter-thymeleaf'
implementation 'org.springframework.boot:spring-boot-starter-web'
testImplementation 'org.springframework.boot:spring-boot-starter-test' //테스트 라이브러리 default로 들어감
}
tasks.named('test') {
useJUnitPlatform()
}
3. JAVA main method 실행해보기
SpringBootApplication이 내장된 웹 서버 tomact으로 띄워진다. (localhost:8080)
+) gralde을 통하지않고 IDE에서 바로 JAVA 실행 설정
preferences > Gradle 검색 > Build and rund using, Run tests using 모두 IntelliJ IDEA로 변경
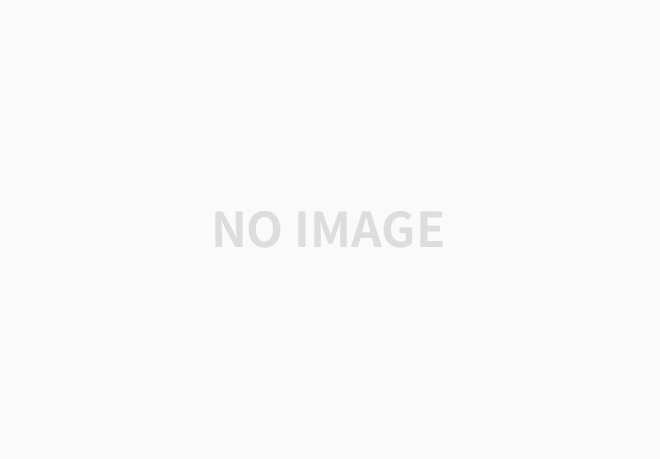
다시 빌드 시도해보면 속도가 빨라졌음을 체감할 수 있다.
4. View단 설정
src/main/resources/static/index.html에 소스코드를 작성하면, 웹 브라우저에서 확인 가능하다.
정적 페이지로 띄우는 단순 작업이다.
* 공식문서는 언제나 적극 활용할 것!
https://docs.spring.io/spring-boot/docs/current/reference/html/index.html
Spring Boot Reference Documentation
The reference documentation consists of the following sections: Legal Legal information. Getting Help Resources for getting help. Documentation Overview About the Documentation, First Steps, and more. Getting Started Introducing Spring Boot, System Require
docs.spring.io
(1) 템플릿 엔진 사용해보기
먼저, controller 패키지를 하나 생성한 후 하위 파일로 자바 클래스를 생성하고 아래와 같이 작성한다.
package com.example.springexample.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
@Controller
public class HelloController {
@GetMapping("hello")
public String Hello(Model model){
model.addAttribute("data","hello");
return "hello";
}
}
- GetMapping: http 통신 중 get 방식
- 예를 들어 위에서 처럼 @GetMapping("hello") 는 "도메인/hello" 로 접속했을 때 Hello 함수를 실행하라는 것과 같은 의미
- model에서 data 속성을 조회해서 그 값을 "hello"로 지정한다.
- return "템플릿명" : resources > templates 에서 hello.html를 찾아서 실행하라. (템플릿 엔진 처리)
- viewResolver(뷰리졸버) : 템플릿의 viewName을 매핑하는 역할.
templates > hello.html 생성
<!doctype html>
<html xmls:th="http://www.thymeleaf.org">
<head>
<title>Hello World</title>
<meta http-equiv="Content-Type" content="text/html"; charset="UTF-8"/>
</head>
<body>
<p th:text="'안녕하세요. '+${data}">안녕하세요</p>
</body>
</html>
- ${data}에 data key값과 매칭되는 value값인 "hello"가 들어온다.
5. 빌드하기
(macOS 기준)
./gradlew build
빌드가 성공적으로 완료되었다면 /build 디렉토리가 생성된 것을 확인할 수 있다.
cd build/libs
해당 경로로 접속하면, jar파일이 말린 것을 확인할 수 있다.
그러면 java -jar 패키지명 을 통해 실행시키면 된다. (로컬에서 띄울 경우, 다른 곳에서 8080포트 사용 여부 확인할 것)
실제로 배포할 때도, 마찬가지로 이 jar파일만 서버에 띄워서 실행시키면 되는 것이다.
*이 글은 인프런에서 제공하는 "스프링 입문 - 코드로 배우는 스프링 부트, 웹 MVC, DB 접근 기술" 강의를 수강하면서 작성한 글입니다.